Submission: Submit your assignment using Wolfware Classic. Remember that 10% of your assignment grade is for correctly submitting your work! For more information about how to correctly submit, see this post on the class website.
Goal: In this assignment you will implement transforms (scaling, rotation, and translation), lighting and texture mapping on 3D objects using a rasterization API (either WebGL or OpenGL).
Grading:
All graphics functionality of this assignment must be completed using a rasterization API (WebGL or OpenGL). Your assignment will be evaluated as follows:
- 10% Properly turn in the assignment.
- 20% Render triangles described in an OBJ file with a basic white material.
- 20% Using shaders, perform Blinn-Phong lighting on the triangles (per-vertex or per-pixel).
- 30% Using shaders, perform texture mapping on the triangles.
- 20% Using shaders, interactively apply transformations to the triangles.
- Participation credit: You can receive participation credit (outside of this assignment) for posting images of your progress, good or bad, on the class forum!
General Notes (read carefully!):
You are free to choose the window size, position of eye, and light position and color. By default, your renderer should choose a view that makes the model visible! Since the assignment requires
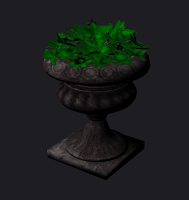
Your program should work with the test cube, along with several several other obj files, a few of which you can find here. Your parser must support texture coordinates for texture mapping.
Two sample OBJ models with texture coordinates and accompanying textures are provided for you to use. You can download them here. Remember, OBJ files specify MTL files, which in turn specify texture files to load. Make sure the paths in each file are correct!
How Your Assignment Will Be Invoked (read extra carefully!):
JS / WebGL: your program must accept a query string parameter specifying the OBJ file to load. The browser will be run with --disable-web-security in order to allow cross origin requests. We will run your assignment using:
myassignment/index.html?objfilepath=[objfilepath]
C / OpenGL: your program must accept a command line argument specifying the OBJ file to load. We will run your assignment using:
myassignment.exe [objfilepath]
Assignment Details:
Part 0: Properly turned in assignment
Use a rasterization API (WebGL or OpenGL) to render triangles loaded from OBJ files. You should see all objects rendered in pure white, with only the silhouette providing details about the object's shape.
Part 2: Render lit, depth buffered triangles
Part 2: Render lit, depth buffered triangles
Using shaders and the rasterization API, perform depth buffering and Blinn-Phong lighting. Blinn-Phong lighting can be calculated in either the vertex shader or fragment shader.
Part 3: Render lit, depth buffered, texture mapped triangles
Load a texture associated with a material specified in the material library file (.mtl). Use this image and the texture coordinates specified in the OBJ file to perform texture mapping on a 3D object which uses the texture mapped material.
By default, you should support the BMP image format although you are free to support additional image formats as extra credit. If you are using C / OpenGL, you may use Windows or D3D11 helper functions to load images. If you are using JavaScript / WebGL, you may use jQuery helper functions to load images.
Part 4: Scaling, Rotation and Translation
Interactively perform transformations (scaling, rotation, and translation) on the rendered triangles, using the keyboard and cursor keys (arrow keys) for input. Transformations must be performed using shaders.Part 3: Render lit, depth buffered, texture mapped triangles
Load a texture associated with a material specified in the material library file (.mtl). Use this image and the texture coordinates specified in the OBJ file to perform texture mapping on a 3D object which uses the texture mapped material.
By default, you should support the BMP image format although you are free to support additional image formats as extra credit. If you are using C / OpenGL, you may use Windows or D3D11 helper functions to load images. If you are using JavaScript / WebGL, you may use jQuery helper functions to load images.
Part 4: Scaling, Rotation and Translation
Let the user transform the rendered object as follows:
- Use "z" to zoom-in/scale-up the object
- Use "x" to zoom-out/scale-down the object
- Use "up-arrow" to move the object in +Y
- Use "down-arrow" to move the object in -Y
- Use "right-arrow" to move the object in +X
- Use "left-arrow" to move the object in -X
- Use "[" to move the object in +Z
- Use "]" to move the object in -Z
- Use "q" to rotate the view in clockwise direction
- Use "w" to rotate the view in anti-clockwise direction
Extra Credit:
Extra credit opportunities include the following, with others possible with instructor approval:
- 2.5% support arbitrarily sized images (and interface windows)
- 2.5% support multiple lights at arbitrary locations
- 2.5% support additional image file formats
- 2.5% implement alpha test in pixel shaders
Arbitrarily sized interface windows
Allow users to resize the interface window, and change the graphics window to match. This should effect every part of your assignment.
Extra credit: Multiple and arbitrarily located lights
Extra credit: Multiple and arbitrarily located lights
Read in an additional lights.txt file that on each line, describes the location and color of a light (use three triples for a light's color: ambient, diffuse and specular). Render the scene with these lights. This should affect every part of your assignment.
Extra credit: Multiple image formats
Extra credit: Multiple image formats
Implement support for loading at least one additional image format beyond BMP (e.g. PNG, JPG, DDS).
Extra credit: Alpha Test
Implement alpha test in the pixel shader. Demonstrate alpha test working with the provided vase model and DDS texture.